Getting Started
Noise Cancellation
C++ Library Documentation
Check out the C++ API here.
Example
The technical integration of Krisp Audio SDK should have correct sequence of functions invocation. The following example shows how to use the exposed functions. For more details, please refer to the sample apps.
try
{
globalInit(L"");
ModelInfo ncModelInfo;
ncModelInfo.path = weightFilePath; // .kef file
NcSessionConfig ncCfg =
{
inRate,
frameDurationMillis,
outRate,
&ncModelInfo,
withStats,
nullptr // Ringtone model cfg for inbound stream case
};
std::shared_ptr<Nc<SamplingFormat>> ncSession = Nc<SamplingFormat>::create(ncCfg);
while(frameAvailable)
{
ncSession->process(inFrame, inFrameSize, outFrame, outFrameSize,
noiseSuppressionLevel, withStats ? &perFrameStats : nullptr);
}
// ncSession is a shared_ptr. Free pointer before globalDestroy()
ncSession.reset();
globalDestroy();
}
catch(std::exception& ex)
{
// Handle exception
}
Background Voice Cancellation
Device Requirements
Implementing the BVC integration requires detecting the connected audio device, matching against the allow/block lists tested by Krisp, and optionally comparing it to the local allow list.
Device List | Description |
---|---|
Krisp allow list | These devices have been tested and approved by Krisp. You may safely enable BVC automatically. |
Krisp block list | These devices have been tested and rejected by Krisp as they showed poor performance and quality. You are not recommended to enable BVC on these devices. |
Local allow list | If the connected device is not in the allow or block list, you may still want to allow the user to enable BVC manually. Then, the app may maintain a local allow list for devices to look up for enabling BVC. |
Recommended Pipeline
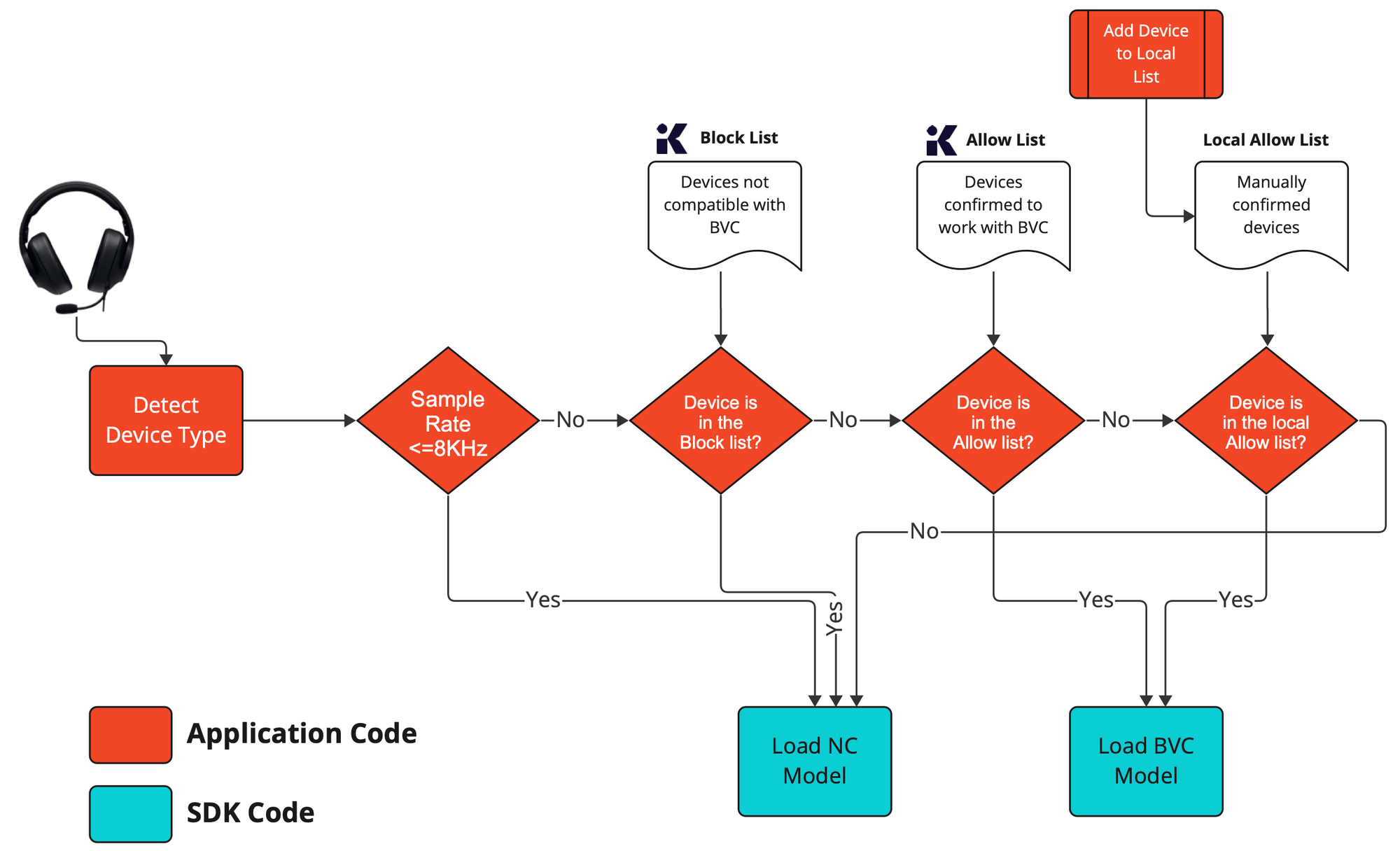
Integration
Below are the high level steps required for technical integration:
SDK initialization | the SDK library should be loaded and initialized before being used. The SDK occupies resources that should be released once the SDK is no longer needed. |
---|---|
Model initialization | Krisp SDK ships with NC and BVC models. Each model has specific requirements for the device and the sampling rate. Each model should be initialized and loaded into the memory before it can be used. |
Session creation | In order to use Krisp Audio SDK to process the sound stream a Session object should be created. The Session creation is coupled to the AI model which should be specified during the session creation process. The session object also requires the specification of the sampling rate and frame duration. |
Frame processing | Frame processing is done using the Session object which works only for the given sampling rate for the given frame duration using the loaded AI model. |
Releasing the resources used by the session | the resources occupied by the session should be released once the session is no longer used. |
Unloading the SDK | the resources loaded by the Krisp Audio SDK should be released once the SDK is no longer needed |
ℹ️ Multiple sessions can be created and used at the same time
ℹ️ Each session can be processed in a different thread on another audio stream
ℹ️ A dummy Session can preload model into memory, while a separate Session could handle the actual stream processing. This approach may be helpful to reduce latency before processing begins, as the second Session will utilize the cached model data preloaded by the first Session and will be created much faster than the first Session.
❗The SDK should be unloaded only after the release of all sessions
Linux Integration
Managing Multithreaded Behavior
By default, the SDK operates in multi-threaded mode to optimize performance and support a higher number of concurrent sessions on a given CPU. The actual number of threads is managed dynamically by the OpenBLAS runtime, typically allowing more sessions to run than the number of available CPU cores.
If your application already processes Krisp Noise Cancellation (NC) in multiple threads, it is recommended to configure the SDK to run in single-threaded mode to prevent excessive thread contention and optimize overall system performance:
export OPENBLAS_NUM_THREADS=1
Updated 3 months ago