WebRTC: Android
Introduction
To integrate the Krisp SDK with WebRTC on both iOS and Android platforms, the Krisp customer integration team introduced the Audio Hook method, leveraging the Audio Processing functionality provided by the WebRTC PeerConnectionFactory implementation. This method enables the creation of a customized Audio Processor and its integration with the PeerConnection during the build process, utilizing the PeerConnectionFactoryBuilder available in the WebRTC framework.
For Android integration, an automated process is employed to generate a JNI (Java Native Interface) bridge, which is then embedded into the WebRTC framework. As an extension, users are provided with the Krisp Java interface that can then be incorporated into their applications. This interface facilitates smooth integration and empowers users to effectively utilize Krisp within their app.
Stream-flow diagram
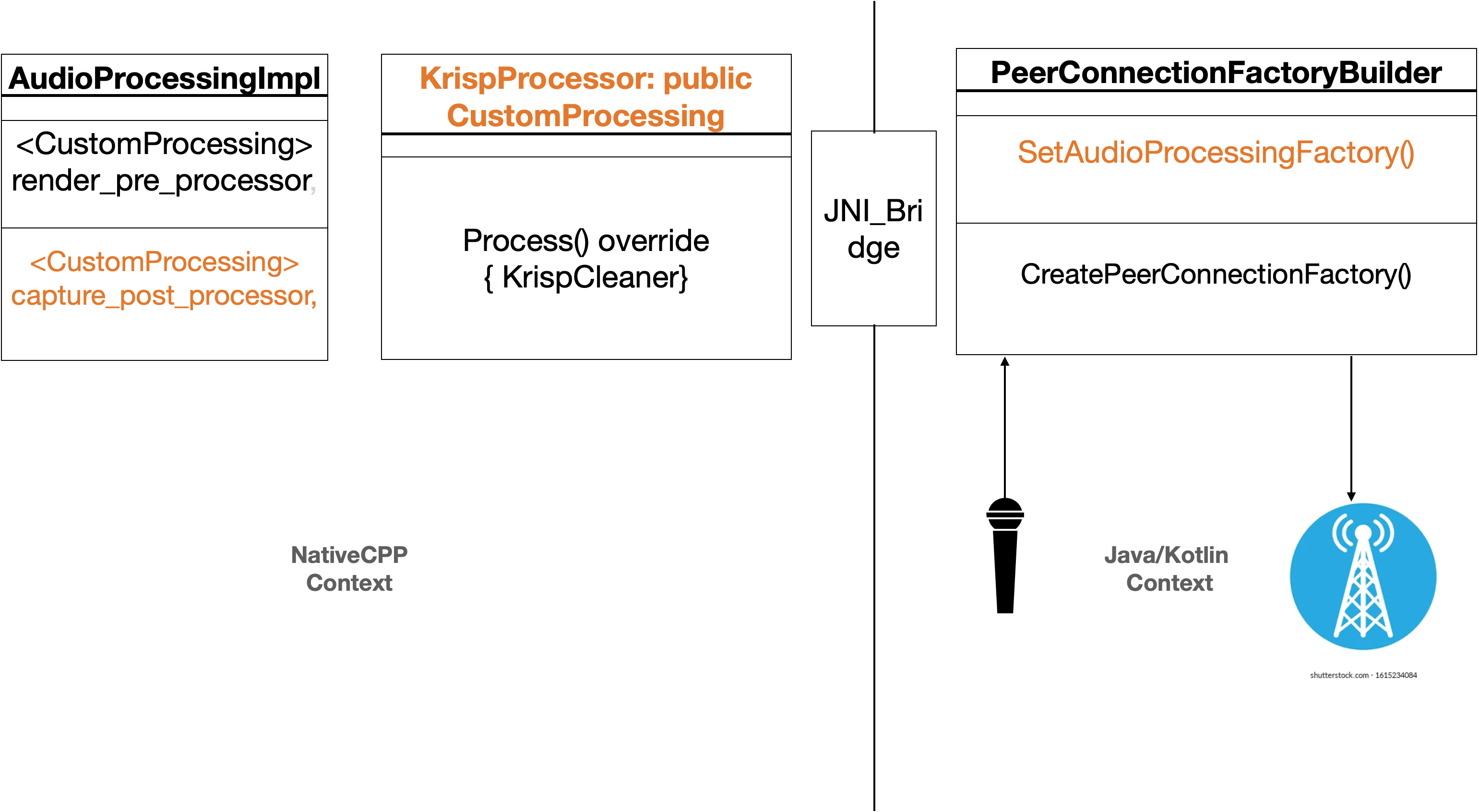
Building WebRTC with Krisp extension for Android
Step 1: Install Google depot_tools.
git clone https://chromium.googlesource.com/chromium/tools/depot_tools.git
Step 2: Add the depot_tools installation root folder to the PATH variable.
export PATH=`pwd`/depot_tools:$PATH
Step 3: Get webrtc sources
fetch --nohooks webrtc_android
gclient sync
cd src
Step 4: Modify sdk/android/BUILD.gn file as follow
- Add krisp_processor library into the dependency list of
rtc_shared_library("libjingle_peerconnection_so")
rtc_shared_library("libjingle_peerconnection_so") {
......
deps = [
...
...
“../../krisp-android-wrapper:krisp_processor”,
]
...
}
- Add krisp_java library into the dependency list of
_dist_jar(“libwebrtc”)
for creating public java modules
dist_jar("libwebrtc") {
...
deps = [
...
...
“../../krisp-android-wrapper:krisp_java”,
]
....
}
Step 5: Clone krisp-android-wrapper directory
git clone [email protected]:krispai/krisp-android-wrapper.git
Step 6: Build libwebrtc.aar
python3 tools_webrtc/android/build_aar.py
Krisp Java interface
KrispAudioProcessingImpl(): Constructs webrtc::AudioProcessing
instance with Krisp processor and returns a pointer to it
val apm = KrispAudioProcessingImpl();
KrispInit(String): pass the model, perform native call and execute krispAudioGlobalInit
, and krispAudioSetModel
//path to device storage where weight.kw is stored
apm.KrispInit("**path/to/krisp/weight.kw");**
KrispAudioBypass(boolean): Bypass KrispProcessing
switch.setOnCheckedChangeListener { buttonView, isChecked ->
if(isChecked) {
apm.KrispDisable(false);
} else {
apm.KrispDisable(true);
}
}
KrispDestroy(): delete webrtc::AudioProcessing
object then call krispAudioNcCloseSession()
and krispAudioGlobalDestroy()
override fun onDestroy() {
apm.KrispDestroy()
}
Integration
Step 1: Import new libwebrtc.aar framework in the app’s gradule file (build.gradule)

Step 2: Add lib-krisp-audio-sdk.so
into jniLibs/<arch-abi>
sub-folder
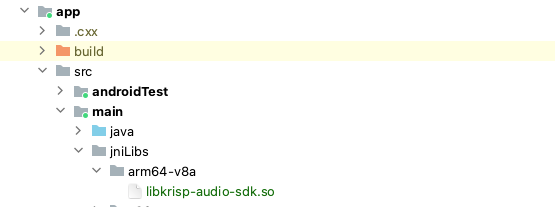
Step3: Use of KrispAudioProcessor in java/kotlin as follows
//imort the header
import org.webrtc.KrispAudioProcessingImpl
// Creating KrispProcessor object
val apm = KrispAudioProcessingImpl();
// Initializing
apm.KrispInit("/path/to/model.kw");
// Creating PeerConnection with Krisp solution
PeerConnectionFactory
.builder()
.setAudioProcessingFactory(apm)
...
.createPeerConnectionFactory()
Updated 8 months ago