Integrate into Native Apps
How to integrate Krisp C-Library into your native app
Krisp SDK for native desktop and mobile applications is a C-library.
Krisp SDKs are deployed and in use globally across more than 200M end-user applications and devices. Krisp SDKs power Krisp's own application in use by enterprises around the world, it also powers voice quality for Discord, RingCentral, Twilio and others.
The C-library is designed to be integrated into native apps running on Windows, Mac, Linux, Android and iOS.
The library can also be integrated directly into WebRTC or other communication frameworks.
C-Library Documentation
Check out the C functions and data structures here.
How to use the library
The following example shows how to use the exposed functions. For more details, please refer to the sample apps.
// Initialize the SDK v6.x
if (krispAudioGlobalInit(workingPath) != 0) {
std::cerr << "Failed to initialize SDK" << std::endl;
return -1;
}
// Initialize the SDK v5.x
if (krispAudioGlobalInit(workingPath, 1) != 0) {
std::cerr << "Failed to initialize SDK" << std::endl;
return -1;
}
// Set the neural network model path
if (krispAudioSetModel(weightConfFilePath)) {
std::cout << "Faied to set the weight file: " << weight_str << std::endl;
return -1;
}
// Create audio session
KrispAudioSessionID noiseCancellerSession = krispAudioNcCreateSession(inputSampleRate,
outputSampleRate,
frameDuration,
modelName);
if (noiseCancellerSession == nullptr) {
std::cerr << "Failed to create noise cancellation session for channel" << std::endl;
return -1;
}
// For every audio frame, call this function to remove noise
krispAudioNcCleanAmbientNoiseInt16(noiseCancellerSession,
pFrameIn,
frameInSize,
pFrameOut, frameOutSize);
// Close the session and uninitalize the SDK
krispAudioNcCloseSession(noiseCancellerSession);
// Uninitalize the SDK
krispAudioGlobalDestroy();
Supported Audio Characteristics
Audio frame durations
10ms, 20ms, 30ms, 40ms
Audio sample rates
8KHz, 16KHz, 32KHz, 44.1KHz, 48KHz, 88.2KHz, 96KHz
Performance
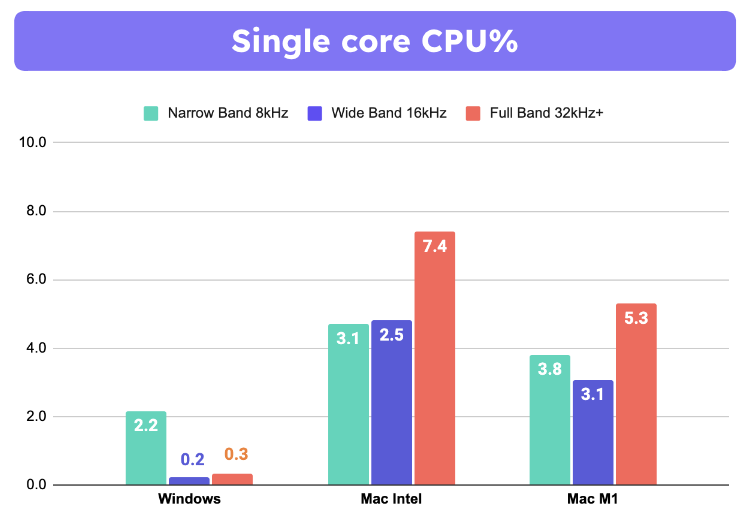
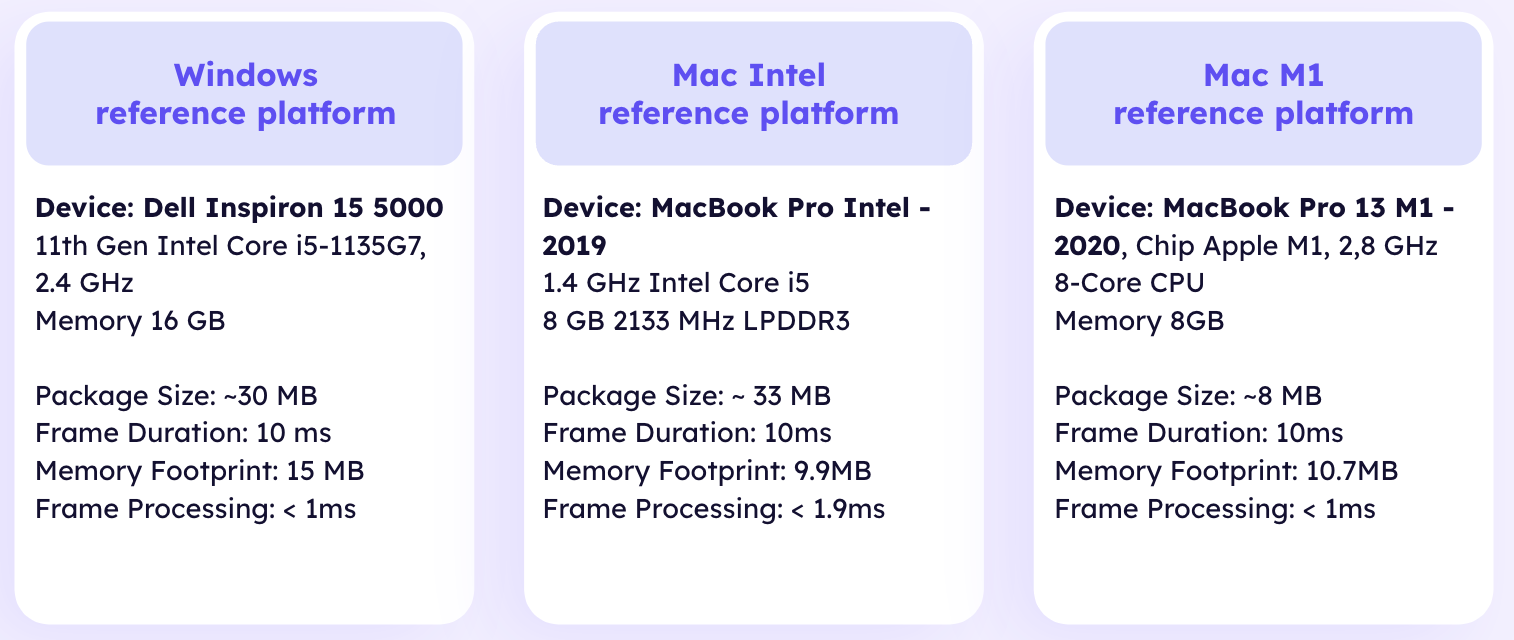
Sample Apps
Check out our Sample apps for Windows, Mac and Linux.
v6.x
v5.x
Third-Party Dependencies
Krisp C-Library has platform-specific dependencies on third-party libraries.
- OpenBLAS libraries are required for the Android platform. These are included in the Krisp SDK archive, which means that all link time references are available out of the box.
- Accelerator libraries are required for iOS and ARM CPU-based MacOS platforms already available on macOS and IOS systems.
- Intel MKL libraries are required for all x86/amd64-based platforms. The library is usually unavailable with the operating system out of the box, which is why you should manually install Intel MKL libraries to use Krisp Audio SDK on all x86/amd64-based platforms.
Mac Intel: Integration with Clang
Description
Krisp SDK for the Mac Intel platform requires Intel MKL libraries that are not packaged with the operating system. The SDK user must install and properly configure Intel MKL libraries.
Library Installation
The following link is available to download and install Intel MKL libraries for the Mac Intel platform.
⬇️ Download MKL
After the installation, the library is expected to be installed in the following location
/opt/intel/oneapi/mkl/latest
ℹ️ Note: Krisp SDK does not depend on this location.
Linker parameters
${MKLROOT}/lib/libmkl_intel_ilp64.a ${MKLROOT}/lib/libmkl_sequential.a ${MKLROOT}/lib/libmkl_core.a -lpthread -lm -ldl
Compiler parameters
-DMKL_ILP64 -m64 -I"${MKLROOT}/include"
where
${MKLROOT} is expected to be set to /opt/intel/oneapi/mkl/latest
Please use the Linker Parameters since Krisp SDK archives need those symbols.
Mac ARM: Integration with Clang
Krisp SDK for the Mac ARM platforms requires the Accelerator library available with the operating system. The following parameters should be used to resolve external symbols during the build properly.
-lblas -lpthread -lm -ldl
Linux Intel: Integration with GCC Compiler
Description
Krisp Audio SDK for Mac Intel platform requires Intel MKL libraries the that are not packaged with the operating system. Follow the directions to install and properly configure Intel MKL libraries.
Installation
Download the package from the link below.
⬇️ Download MKL
On Linux Intel, it could be located at the following location.
$HOME/intel/mkl
ℹ️ Note: Krisp SDK does not depend on this location.
Further ${MKLROOT} variable will refer to the installation location.
Compiler parameters
-DMKL_ILP64 -m64 -I"${MKLROOT}/include"
Linker parameters
Wl,--start-group ${MKLROOT}/lib/intel64/libmkl_intel_ilp64.a ${MKLROOT}/lib/intel64/libmkl_sequential.a ${MKLROOT}/lib/intel64/libmkl_core.a -Wl,--end-group -lpthread -lm -ldl
Windows: Integration with Visual Studio Compiler
Before importing Krisp Audio SDK header and any other define the following C/C++ macros
Compiler parameters
#define _ITERATOR_DEBUG_LEVEL 0
// before including any other header
// #include <vector>
#define KRISP_AUDIO_STATIC
// before including krisp-audio-sdk.hpp
#include <krisp-audio-sdk.hpp>
Instead of defining C/C++ macros in the source you can pass it to the compiler per your preference.
KRISP_AUDIO_STATIC macro is needed to set up proper link to symbols in the Krisp Audio SDK static library.
_ITERATOR_DEBUG_LEVEL=0 is required to keep the example codebase compatible with Krisp Audio SDK static library.
Library Installation
The following link is available to download and install Intel MKL libraries for the Windows x86/amd64 platform.
After the installation, it should be available in the following location
C:\Program Files (x86)\Intel\oneAPI\mkl\latest
Further, the ${MKLROOT} environment variable is supposed to refer to this directory
Where headers should be located in
${MKLROOT}\include
32-bit libraries should be located in
${MKLROOT}\lib\ia32
64-bit libraries should be located in
${MKLROOT}\lib\intel64
Microsoft Visual Studio Toolset 64 bit build
Compiler options
/DMKL_ILP64 -I"%MKLROOT%\include”
Linker options
mkl_intel_ilp64.lib mkl_sequential.lib mkl_core.lib
Microsoft Visual Studio Toolset 32 bit build
Compiler options
I"%MKLROOT%\include”
Linker options
mkl_intel_c.lib mkl_sequential.lib mkl_core.lib
Intel CPU-based systems
You can use the following Web resource to choose a different way of using MKL libraries. This could be useful if you need to use a specific compiler on a platform that is not listed in this page. Please consider that Intel MKL libraries are required by Krisp Audio SDK for all Intel CPU based platforms only.
The resource will provide platform and compiler-specific options for a chosen integration type. You are more than welcome to experiment if you need to.
Select Intel® product: oneMKL 2022
Select OS: macOS
Select programming language: C/C++
Select compiler: Clang
Select architecture: Intel® 64
Select dynamic or static linking: Static
Select interface layer: C API with 64-bit integer
Select threading layer: Sequential
Link with Intel® oneMKL libraries explicitly: checked
The specified selection is supposed to produce the following output
This will produce the following compiler and linker parameters
Compiler parameters
-DMKL_ILP64 -m64 -I"${MKLROOT}/include"
Linker parameters
Wl,--start-group ${MKLROOT}/lib/intel64/libmkl_intel_ilp64.a ${MKLROOT}/lib/intel64/libmkl_sequential.a ${MKLROOT}/lib/intel64/libmkl_core.a -Wl,--end-group -lpthread -lm -ldl
Android
OpenBLAS
is used and integrated into Krisp Audio SDK archive libraries. There is no third-party dependency, and no specific options should be specified. This means that all third-party references are resolved out of the box.
iOS
iOS's Accelerator
library is used, which is available on the operating system. No other third-party dependency here.
Updated over 1 year ago