Integrate into Web Apps
How to integrate Krisp SDK into your web app
Krisp SDK is the only robust Javascript-based Noise Cancellation SDK available for browsers. Running audio AI inside the browsers is challenging for various reasons.
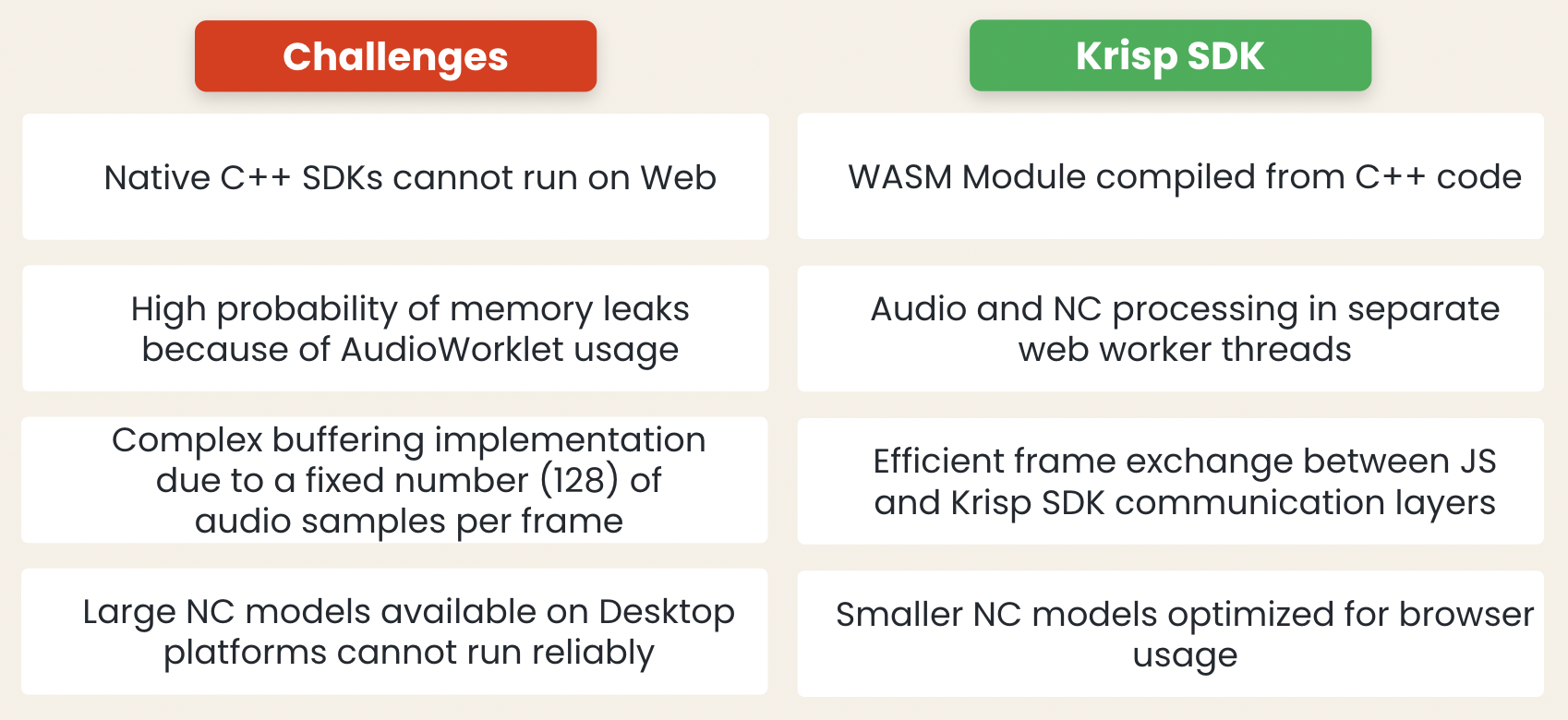
Example Code
Connect the noise filter to an element on your page
import KrispSDK from "/dist/krispsdk.mjs";
const audioContext = new AudioContext();
const krispSDK = new KrispSDK({
params: {
models: { model8: "{MODEL_PATH}", model16: "{MODEL_PATH}", model32: "{MODEL_PATH}" },
},
callbacks: {},
});
/* Get the stream from browser */
navigator.mediaDevices.getUserMedia({audio: true}).then(async stream => {
await audioContext.resume();
await krispSDK.init();
/* Create audio filter for noise cancellation and enable it */
const filterNode = await krispSDK.createNoiseFilter(audioContext, () => {
filterNode.enable();
});
/* Create source and destination streams and connect the filter to them */
const source = audioContext.createMediaStreamSource(stream);
const destination = audioContext.createMediaStreamDestination();
source.connect(filterNode);
filterNode.connect(destination);
/* Connect destination stream to an audio Element and play it */
const audioElement = document.createElement("audio");
audioElement.srcObject = destination.stream;
audioElement.play();
})
Sample Web Apps
The following sample apps are currently available for further exploration:
Audio Pipeline and Processing
Krisp Javascript SDK is integrated with WebRTC and Web Audio as a noise audio filter.
The noise-canceling neural network is built on WASM for high performance.
The SDK receives audio buffers in chunks and processes them in a dedicated worker to offload the main thread.
Performance
- Package Size: 12MB
- Memory Footprint: ~15 MB
- Frame Processing: 1.5-2ms
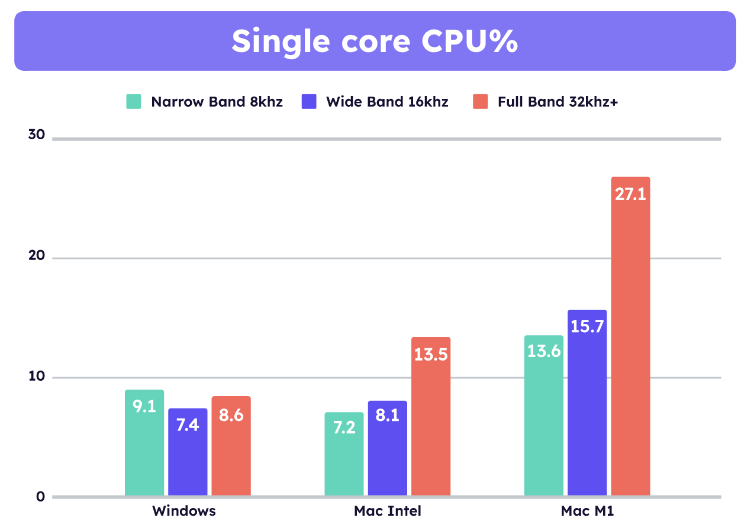
Benchmarks conducted for JS SDK v1.0.8 with Chrome v108 on Mac M1 and Chrome v109 on Windows and Mac Intel on the same reference computers used for Desktop. On Mac M1 Krisp SDK runs ~85% on high-efficiency cores resulting in relatively higher CPU% but ensuring better battery performance.
Enabling Shared Array Buffer results in decrease in CPU performance.
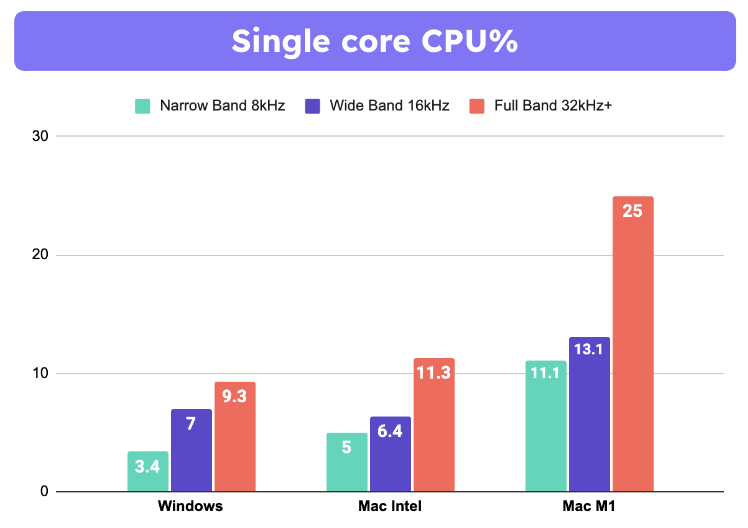
Measurements conducted for JS SDK v1.0.8 with Shared Array Buffer enabled on the same reference devices and browser versions
Important Notes
- CPU% is generally higher in JS SDK compared to native SDK. This is expected as the code runs in the browser in different worker threads, which provides improved stability and eliminates memory leaks.
- Krisp JS SDK is not supported on mobile browsers. Krisp recommends using Native iOS and Android SDKs for mobile applications.
- Safari browser is not supported due to inconsistent performance across different versions caused by internal bugs in Safari proper.
Updated over 1 year ago